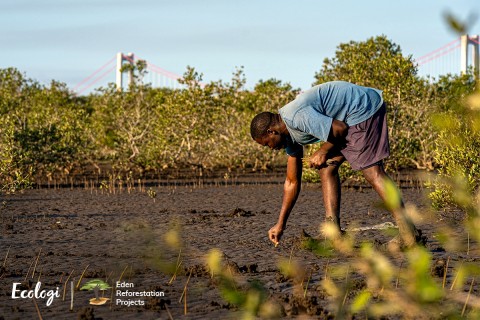
Planting Trees with Code
In September 2021, A Digital signed up to Ecologi to support tree planting and carbon offset projects to make a small contribution towards fighting climate change.
Everywhere we look we're seeing increasingly urgent calls to stem the tide of climate change and the wide ranging impact we're having on our environment. Living and working on the edge of the Lake District National Park which is also a UNESCO World Heritage Site, we're acutely aware of how fortunate we are to be in such an incredible location surrounded by a rich and diverse landscape.
Ecologi is a subscription platform that allows businesses and individuals to offset the carbon footprint of their team, either by planting trees, or supporting a wide range of certified carbon offsets. The platform funds tree-planting initiatives, such as the Eden Reforestation Project in Madagascar, and other carbon reduction schemes around the world, supporting the United Nations Sustainable Development Goals.
Founded by three friends in Bristol, UK, Ecologi’s mission is to help individuals and businesses combat climate change and restore nature by funding reforestation projects as well as climate solutions to offset carbon. Since their launch 20 months ago, they have planted over 22 million trees and offset 300,000 tonnes of CO2.
As a small business, we're well aware that our individual contribution is small, but collectively, the vast number of small businesses have enormous power to impact significant change with small measures.
As a marketing and technology company, we're also uniquely positioned to support and influence more sustainable behaviours. Technology is often out of site and therefore out of mind. While we're seeing people take issue with obvious environmental issues such as transport and energy, technology has so far remained largely under the radar. Yet data centres are some of the most demanding energy consumers on earth, so we wanted to play our part to offset some of the data the we're storing in them.
How are we planting trees?
The beauty of the Ecologi platform is that it integrates seamlessly with technology.
All the code we write during a website project gets stored in a version controlled repository which keeps it safe, and prevents it from being overwritten by other contributors on our team.
We 'commit' code to the repository (or repo for short), so we thought for every code commit, we can plant a tree. Larger projects will have a higher number of commits, so they will see more trees planted. Pretty cool huh?
Some of our projects will easily hit 1,000 commits over the course of several months, so that should take us well on our way to a target of 10,000 trees to be planted over the next year.
Woah! You've lost me - how else can I plant trees with tech?
Well, pretty easily as it happens. Ecologi connects to Zapier, so you can trigger tree planting with no code required from all sorts of different events such as receiving a Stripe Payment, creating an invoice in Xero or when someone joins your MailChimp mailing list. The list of options is endless, so it's easy to contribute and reduce your own carbon footprint.
Sample Code for Developers
If you're a developer that likes the idea of planting trees with code, the following PHP sample is a webhook script to plant a tree with Ecologi on each commit (or push to be more specific) from either a GitHub or BitBucket repository.
As we use a combination of both BitBucket and GitHub repositories for our projects, the sample code below will work with both platforms. The request headers and data payloads from each platform differ slightly, and of course you'll need to replace the dummy authorization token with your API key from Ecologi.
Once you've added the code below to your server, you can set the URL as the webhook on your repo settings.
You're welcome to use it in your own projects, although as usual, it's provided 'as is' with no support or warranty.
<?php
/**
* Web hook processor to capture a GitHub or BitBucket commit and plant a tree with Ecologi.
*
* For TESTING, set an extra header with any value, eg. Testing: Yes
*
* BitBucket Webhook Reference: https://support.atlassian.com/bitbucket-cloud/docs/event-payloads/#EventPayloads-Push
* GitHub Webhook Reference: https://docs.github.com/en/developers/webhooks-and-events/webhooks/webhook-events-and-payloads#push
* Ecologi API Reference: https://docs.ecologi.com/
* Test a sample payload with headers at https://reqbin.com/
*
* @author Andrew Armitage A Digital @adigital_uk
*
*
* The MIT License (MIT)
*
* Copyright (c) A Digital
*
* Permission is hereby granted, free of charge, to any person obtaining a copy of
* this software and associated documentation files (the "Software"), to
* deal in the Software without restriction, including without limitation the
* rights to use, copy, modify, merge, publish, distribute, sublicense, and/or
* sell copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
//check headers
$headers = getallheaders();
//look for the header so we can check the source and validate request
if(isset($headers['X-Event-Key'])) {
$source = "bitbucket";
$eventKey = $headers['X-Event-Key']; //repo:push
}
elseif(isset($headers['X-Github-Event'])) {
$source = "github";
$eventKey = $headers['X-Github-Event']; //push
}
//set an optional header for testing purposes
if(isset($headers['Testing'])) {
//this is the value to add to the Ecologi Curl post fields
$test = ",\"test\": true";
}
//check the header matches push event header
if($eventKey == "repo:push" || $eventKey == "push") {
//we're good to go and read in the data so decode the JSON payload
$payload = json_decode(file_get_contents('php://input'));
//if the payload isn't empty, we need to check the structure of the JSON
if(!empty($payload)) {
//check the source and look for a valid push object
if($source == "bitbucket" && isset($payload->push)) {
//grab the repo name so we can submit this to Ecologi
$client = $payload->repository->project->name;
}
//check the source and look for a valid push object
elseif($source == "github" && isset($payload->pusher)) {
//grab the repo name so we can submit this to Ecologi
$client = $payload->repository->owner->name;
}
else {
//something is missing so stop here
echo "Error: Can't find a valid push from " . ucfirst($source) . " or unable to get the client name. Do you need to check the repo source?";
exit;
}
//now we can build the Curl request for Ecologi
$curl = curl_init();
//set options
curl_setopt_array($curl, [
CURLOPT_URL => "https://public.ecologi.com/impact/trees",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => "{\"number\":1,\"name\":\"".$client."\"".$test."}",
CURLOPT_HTTPHEADER => [
"Content-Type: application/json",
"authorization: 000-000-000-000"
],
]);
//get the response
$response = curl_exec($curl);
$err = curl_error($curl);
//close the connection
curl_close($curl);
if ($err) {
echo "Curl Error #:" . $err;
}
else {
echo $response;
}
}
//no payload received, nothing to do
else {
echo "Error: JSON payload is empty.";
exit;
}
}
else {
echo "Error: No value found or incorrect value for Event Key header.";
exit;
}
?>
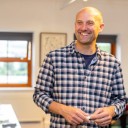
Andrew Armitage
Andrew is the founder of multi-award winning A Digital and believes that technology should be an enabler, making a positive impact on the way people live and work.